Bindings expressions reference
Here you can find the reference for the bindings expressions you can add to the bindings of your nodes, styles, and state managers. See Using styles and State manager.
You can create bindings after you add the Bindings property to a node. Blue type marks the properties that are controlled by a binding. The properties you bind override the properties set in the Properties.
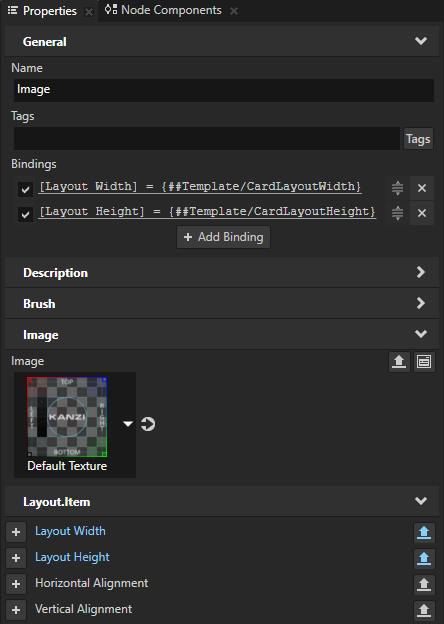
When creating bindings, keep in mind that:
- Only bindings to similar data types are valid. For example, you can bind only color to color, vector2 to vector2, and so on.
- Binding takes the value of the last expression, whether it is an assignment, unary or binary operation, or just a constant value or variable itself.
- In bindings you can cast strings between the four fundamental types: integer, float, boolean, and string. Casts between integer and float are implicit and depend on the type of the property that uses the value. Casts to and from string are explicit.
See Using bindings and Troubleshooting bindings.
Syntax
# (comments)
Use a hash at the beginning of every line that contains a comment. You can use any sequence of characters in comments.
# This is a comment, so you can describe your binding expressions
# Calculate the value of A
A = (2 + 4) / 3
() (parentheses)
Use parentheses to group and contain expressions and parameters, and control the order of execution.
# Containing expressions: calculate the modulo of two values
MOD(23, 27)
# Grouping expressions: first add 2 and 4, then divide the result by 3,
# and return 2
A = (2 + 4) / 3
# First add the FOV property to the Render Transformation Scale X property field,
# then divide the result of the multiplication by 2
({../Camera/Fov} + {../Box/RenderTransformation}.ScaleX) / 2
= (assign)
Assigns a value to a variable.
Syntax
|
var = value
|
Parameters
|
var
|
any valid variable name |
value
|
any supported variable value |
|
Examples
|
# Assigns the value 2.0 to the variable 'A'
A = 2.0 # Assigns the value 4.0 to the variable 'B'
B = 4.0
|
Operators
+ (addition)
Adds two or more values, or combines strings into one.
Syntax
|
value1 + value2
|
Parameters
|
value1
|
color, int, float, string, Vector2, Vector3, Vector4, or boolean: augend |
value2
|
color, int, float, string, Vector2, Vector3, Vector4, or boolean: addend |
|
Returns
|
the same type as parameters, except if one of parameters is float, it returns float |
Examples
|
A = 2.0
B = 4.0
# Returns 6.0
A + B
|
- (subtraction)
Subtracts the value of the second parameter from the value of the first parameter. As a negation operator, it returns the result equivalent to multiplying the value by -1.
Syntax
|
value1 - value2
|
Parameters
|
value1
|
color, int, float, string, Vector2, Vector3, Vector4, or boolean: minuend |
value2
|
color, int, float, string, Vector2, Vector3, Vector4, or boolean: subtrahend |
|
Returns
|
the same type as parameters, except if one of parameters is float, it returns float |
Examples
|
A = 2.0
B = 4.0
# Returns -2.0
A - B
|
* (multiplication)
Multiplies the values of parameters.
Syntax
|
value1 * value2
|
Parameters
|
value1
|
color, int, float, Vector2, Vector3, Vector4, or boolean: multiplicand |
value2
|
color, int, float, Vector2, Vector3, Vector4, or boolean: multiplier |
Note that only one of the parameters can be boolean, but not both.
|
Returns
|
the same type as parameters, except:
- If one of parameters is a float, it returns float
- If one of parameters is boolean, it returns int, or float if at least one parameter is float
|
Examples
|
A = 2.0
B = 4.0
# Returns 8.0
A * B
|
/ (division)
Divides the value of the first parameter by the value of the second parameter.
Syntax
|
value1 / value2
|
Parameters
|
value1
|
color, int, float, Vector2, Vector3, Vector4, or boolean: dividend |
value2
|
color, int, float, Vector2, Vector3, Vector4, or boolean: divisor |
Note that only one of the parameters can be boolean, but not both.
|
Returns
|
the same type as parameters, except:
- If one of parameters is a float, it returns float
- If one of parameters is boolean, it returns int, or float if at least one parameter is float
|
Examples
|
A = 2.0
B = 4.0
# Returns 0.5
A / B
|
Casting
Casts between integer and float are implicit and depend on the type of the property that uses the value. Casts to and from string are explicit.
Integer
Converts a value to an integer. Casts between integer and float are implicit and depend on the type of the property that uses the value. Casts to and from string are explicit.
Syntax
|
INT(value)
|
Parameters
|
value
|
float, boolean, string |
|
Returns
|
int |
Examples
|
# Explicitly converts the string "5" to an integer, adds it to the integer 5,
# and assigns the result to the variable A. Returns integer 10.
A = 5 + INT("5") # Implicitly converts boolean value True to integer, adds it to the integer 5,
# and assigns the result to the variable A. Returns integer 6.
B = 5 + True # Explicitly converts float 5.5 to an integer, adds it to the integer and
# assigns the result to the variable C. Returns integer 7.
C = 2 + INT(5.5)
|
Float
Converts a value to a float. Casts between integer and float are implicit and depend on the type of the property that uses the value. Casts to and from string are explicit.
Syntax
|
FLOAT(value)
|
Parameters
|
value
|
int, boolean, string |
|
Returns
|
float |
Examples
|
# Converts the string "5" to a float, adds it to the integer 5,
# and assigns the result to the variable A. Returns float 10.000000.
A = 5 + FLOAT("5") # Implicitly converts boolean value True to float, adds it to the float 5.1,
# and assigns the result to the variable A. Returns float 6.1.
B = 5.1 + True
|
Boolean
Converts a value to a boolean. Casts between integer and float are implicit and depend on the type of the property that uses the value. Casts to and from string are explicit.
Syntax
|
BOOL(value)
|
Parameters
|
|
Returns
|
bool |
Examples
|
# Converts the boolean value "True" to integer 1, adds it to the integer 41,
# and assigns the result to the variable A. Returns integer 42.
A = 41 + BOOL("True")
|
String
Converts a value to a string. Casts between integer and float are implicit and depend on the type of the property that uses the value. Casts to and from string are explicit.
Syntax
|
STRING(value)
|
Parameters
|
value
|
int, float, boolean |
|
Returns
|
string |
Examples
|
# Converts the integer 5 to a string, concatenates it to the string
# "Five is written as ", and assigns the result to the variable B.
# Returns string "Five is written as 5".
A = "Five is written as " + STRING(5) # Converts the value of the variable A to a string, concatenates it
# to the string "Number of fingers on two hands is ", and assigns the result
# to sthe variable B. Returns string "Number of fingers on two hands is 10".
A = 10
C = "Number of fingers on two hands is " + STRING(A) # Converts the value of the float property Float to a string rounded
# to one decimal place. To show two decimal places replace 10 with 100,
# to show three decimal places replace 10 with 1000, and so on.
a = INT(FLOOR({@../Float}))
b = INT(10*({@../Float} - a))
STRING(a) + "." + STRING(b)
|
Functions
Absolute value
Calculates the absolute value of a number or a variable. The absolute value of a number is always positive.
Syntax
|
ABS(value)
|
Parameters
|
value
|
color, int, float, Vector2, Vector3, Vector4: number to compute |
|
Returns
|
the same type as parameter |
Examples
|
# Returns 5.2
ABS(-5.2)
|
Animate
Binds a property value to a piecewise function that you define in an Animation Data item. See Using piecewise functions in bindings.
Animate
takes two arguments: the property to which you are binding the Animation Data item, and the resource ID or kzb URL of the Animation Data item where you define the piecewise function that you want to use to set the value of the bound property.
When you use a resource ID, you have to place the Animation Data item to a resource dictionary where the node that contains the binding can access it. See Using resource dictionaries.
For example, you can use the Animate
function to set the needle in a gauge to move faster between values 0 and 100 than it does for values larger than 100.
Syntax
|
Animate(property, "animationDataResource")
|
Parameters
|
property
|
path and name of the property you want to use to move along the animation curve instead of time
|
animationDataResource
|
resource ID or kzb URL of the Animation Data item the animation curve of which you want to use to set the value of the bound property |
|
Examples
|
# Uses the Speed property to move along the animation curve
# of the Animation Data item with the resource ID Speed curve.
Animate({@./Speed}, "Speed curve") # Uses the Speed property to move along the animation curve
# of the Animation Data item with the kzb URL Speed curve.
Animate({@./Speed}, "kzb://cluster/Animation Data/Speed curve")
|
Ceil
Calculates the closest integer value that is greater than or equal to the value of the parameter.
Syntax
|
CEIL(value)
|
Parameters
|
value
|
color, int, float, Vector2, Vector3, Vector4: number to compute |
|
Returns
|
the same type as parameter |
Examples
|
# Returns 3.0
CEIL(2.06) # Returns 16.0
CEIL(15.92) # Returns -2
CEIL(-2.06)
|
Clamp
Constrains a value to lie between two values. CLAMP
returns the same value as MIN(MAX(value, low), high)
.
Syntax
|
CLAMP(low, high, value)
|
Parameters
|
low
|
int or float: the lower end of the range to constrain the value |
high
|
int or float: the higher end of the range to constrain the value |
value
|
int or float: the value to constrain |
|
Returns
|
float if any of the parameters is float, otherwise int |
Examples
|
# Returns 1
CLAMP(1, 4, 0.5)
# Returns 4
CLAMP(-3, 8, 4)
|
CreateRotation
Creates rotation in either the Layout Transformation or the Render Transformation property using the quaternion data type. The quaternion data type is used for rotation property fields. See CreateRotationX, CreateRotationY, CreateRotationZ, Rotate, RotateX, RotateY, RotateZ.
Syntax
|
CreateRotation(x, y, z)
|
Parameters
|
x
|
int, or float: the rotation on the X axis in degrees |
y
|
int, or float: the rotation on the Y axis in degrees |
z
|
int, or float: the rotation on the Z axis in degrees |
|
Returns
|
quaternion |
Examples
|
# Rotates the bound node:
# - 10 degrees on the X axis
# - 40 degrees on the Y axis
# - 50 degrees on the Z axis
CreateRotation(10, 40, 50) # Rotates the bound node:
# - 30.5 degrees on the X axis
# - 80 degrees on the Y axis
# - 46.5 degrees on the Z axis
CreateRotation(30.5, 80, 46.5)
|
CreateRotationX
Creates rotation in the Layout Transformation or the Render Transformation property on the X axis using quaternion data type. The quaternion data type is used for rotation property fields. See CreateRotation, CreateRotationY, CreateRotationZ, Rotate, RotateX, RotateY, RotateZ.
Syntax
|
CreateRotationX(x)
|
Parameters
|
x
|
int, or float: the rotation on the X axis in degrees |
|
Returns
|
quaternion |
Examples
|
# Rotates the bound node 55 degrees on the X axis.
CreateRotationX(55)
|
CreateRotationY
Creates rotation in the Layout Transformation or the Render Transformation property on the Y axis using quaternion data type. The quaternion data type is used only for rotation property fields. See CreateRotation, CreateRotationX, CreateRotationZ, Rotate, RotateX, RotateY, RotateZ.
Syntax
|
CreateRotationY(y)
|
Parameters
|
y
|
int, or float: the rotation on the Y axis in degrees |
|
Returns
|
quaternion |
Examples
|
# Rotates the bound node 55 degrees on the Y axis.
CreateRotationY(55) # Rotates the bound node 16.5 degrees on the Y axis.
CreateRotationY(16.5)
|
CreateRotationZ
Creates rotation in the Layout Transformation or the Render Transformation property on the Z axis using quaternion data type. The quaternion data type is used only for rotation property fields. See CreateRotation, CreateRotationX, CreateRotationY, Rotate, RotateX, RotateY, RotateZ.
Syntax
|
CreateRotationZ(z)
|
Parameters
|
z
|
int, or float: the rotation on the Z axis in degrees |
|
Returns
|
quaternion |
Examples
|
# Rotates the bound node 33 degrees on the Z axis.
CreateRotationZ(33) # Rotates the bound node 5.5 degrees on the Z axis.
CreateRotationZ(5.5)
|
ExtractEulerX
Extracts the X Euler angle from the Layout Transformation or the Render Transformation properties. You can use ExtractEulerX
for rotation property fields. ExtractEulerX
takes a quaternion data type value as a parameter and returns float data.
When you set an angle in an SRT3D property, the angle is stored in quaternion data type. Because the ExtractEulerX
function extracts the angle from the quaternion, the returned Euler angle is not the same as the angle originally set in the SRT3D property. Both angles define the same rotation.
See ExtractEulerY, ExtractEulerZ, CreateRotation, CreateRotationX, CreateRotationY, Rotate, RotateX, RotateY, RotateZ.
Syntax
|
ExtractEulerX(rotationField)
|
Parameters
|
rotationField
|
rotation property field of either the Layout Transformation or the Render Transformation property |
|
Returns
|
float |
Examples
|
# Extracts the X Euler angle from a rotation property field
# of the Layout Transformation property.
ExtractEulerX({@./Node3D.LayoutTransformation}.Rotation) # Extracts the X Euler angle from the rotation property field
# of the Layout Transformation property and binds the value
# of the X Euler angle to the Rotation Y property field.
a = ExtractEulerX({@./Node3D.LayoutTransformation}.Rotation)
CreateRotation(0, a, 0)
|
ExtractEulerY
Extracts the Y Euler angle from the Layout Transformation or the Render Transformation properties. You can use ExtractEulerY
for rotation property fields. ExtractEulerY
takes a quaternion data type value as a parameter and returns float data.
When you set an angle in an SRT3D property, the angle is stored in quaternion data type. Because the ExtractEulerX
function extracts the angle from the quaternion, the returned Euler angle is not the same as the angle originally set in the SRT3D property. Both angles define the same rotation.
See ExtractEulerX, ExtractEulerZ, CreateRotation, CreateRotationX, CreateRotationY, Rotate, RotateX, RotateY, RotateZ.
Syntax
|
ExtractEulerY(rotationField)
|
Parameters
|
rotationField
|
rotation property field of either the Layout Transformation or the Render Transformation property |
|
Returns
|
float |
Examples
|
# Extracts the Y Euler angle from a rotation property field
# of the Layout Transformation property.
ExtractEulerY({@./Node3D.LayoutTransformation}.Rotation)
|
ExtractEulerZ
Extracts the Z Euler angle from the Layout Transformation or the Render Transformation properties. You can use ExtractEulerZ
for rotation property fields. ExtractEulerZ
takes a quaternion data type value as a parameter and returns float data.
When you set an angle in an SRT3D property, the angle is stored in quaternion data type. Because the ExtractEulerX
function extracts the angle from the quaternion, the returned Euler angle is not the same as the angle originally set in the SRT3D property. Both angles define the same rotation.
See ExtractEulerX, ExtractEulerY, CreateRotation, CreateRotationX, CreateRotationY, Rotate, RotateX, RotateY, RotateZ.
Syntax
|
ExtractEulerZ(rotationField)
|
Parameters
|
rotationField
|
rotation property field of either the Layout Transformation or the Render Transformation property |
|
Returns
|
float |
Examples
|
# Extracts the Z Euler angle from a rotation property field
# of the Layout Transformation property.
ExtractEulerZ({@./Node3D.LayoutTransformation}.Rotation)
|
Floor
Calculates the closest integer value that is less than or equal to the value of the parameter.
Syntax
|
FLOOR(value)
|
Parameters
|
value
|
color, int, float, Vector2, Vector3, Vector4: number to compute |
|
Returns
|
the same type as parameter |
Examples
|
# Returns 0.0
FLOOR(0.8) # Returns 1.0
FLOOR(1.5)
# Returns 15.0
FLOOR(15.92)
|
Linear step
Performs linear interpolation between two values. LINEARSTEP
returns the same value as CLAMP(0, 1, (value - low) / (high - low))
.
Syntax
|
LINEARSTEP(low, high, value)
|
Parameters
|
low
|
int or float: the lower end of the linear function |
high
|
int or float: the higher end of the linear function |
value
|
int or float: the value to constrain |
|
Returns
|
float if one of the parameters is float, otherwise int:
- 0.0 if
value is less than or equals low
- 1.0 if
value is greater than or equals high
- Otherwise
(value - low) / (high - low)
|
Examples
|
# Returns 0.0
LINEARSTEP(1, 4, 0.5)
# Returns 0.64 (7/11)
LINEARSTEP(-3, 8, 4.0)
|
Max
Determines the larger of the two values and returns the larger value.
Syntax
|
MAX(value1, value2)
|
Parameters
|
value1
|
int, float, Vector2, Vector3, Vector4, or boolean: the first number to compare |
value2
|
int, float, Vector2, Vector3, Vector4, or boolean: the second number to compare |
Note that only one of the parameters can be boolean, but not both.
|
Returns
|
the same type as parameters, float if one of parameters is float, otherwise int |
Examples
|
# Returns 5
MAX(2, 5)
# Returns -2.1
MAX(-10, -2.1)
|
Min
Determines the smaller of the two values and returns the smaller value.
Syntax
|
MIN(value1, value2)
|
Parameters
|
value1
|
int, float, Vector2, Vector3, Vector4, or boolean: the first number to compare |
value2
|
int, float, Vector2, Vector3, Vector4, or boolean: the second number to compare |
Note that only one of the parameters can be boolean, but not both.
|
Returns
|
the same type as parameters, float if one of parameters is float, otherwise int |
Examples
|
# Returns 2
MIN(2, 5)
# Returns -10
MIN(-10, -2.1)
|
Mix
Performs a linear interpolation between two values using a value to weight between them. Kanzi computes the result using this function: (end - start) * weight + start
.
Syntax
|
MIX(start, end, weight)
|
Parameters
|
start
|
int or float: the start of the interpolation range |
end
|
int or float: the end of the interpolation range |
weight
|
int or float: the value used for interpolation between start and end |
|
Returns
|
float if one of parameters is float, otherwise int |
Examples
|
# Returns 2.5
MIX(1, 4, 0.5)
# Returns 0.5
MIX(-1, -4, -0.5)
|
Modulo
Calculates the modulo that is the remainder when one number is divided by another using the mathematical modulo congruence function.
See Remainder.
Syntax
|
MOD(value1, value2)
|
Parameters
|
value1
|
int, float, Vector2, Vector3, Vector4, or boolean: the first number to compare: dividend |
value2
|
int, float, Vector2, Vector3, Vector4, or boolean: the first number to compare: divisor |
Note that only one of the parameters can be boolean, but not both.
|
Returns
|
the same type as parameters, float if one of parameters is float, otherwise int |
Examples
|
# Returns 3
MOD(13, 5)
# Returns 2
MOD(-13, 5)
|
Power
Calculates exponential expressions. It is an efficient way for multiplying numbers by themselves.
Syntax
|
POW(n, e)
|
Parameters
|
n
|
int, float, color, Vector2, Vector3, Vector4: base of the exponential expression |
e
|
int, float, or boolean: power by which to raise the base |
|
Returns
|
the same type as parameter n , except for int it returns float |
Examples
|
# Is equivalent to 2*2*2*2*2 and returns 32
POW(2, 5)
|
Remainder
Calculates the remainder when one number is divided by another rounded towards zero.
See Modulo.
Syntax
|
REM(value1, value2)
|
Parameters
|
value1
|
int, float, Vector2, Vector3, Vector4, or boolean: the first number to compare: dividend |
value2
|
int, float, Vector2, Vector3, Vector4, or boolean: the first number to compare: divisor |
Note that only one of the parameters can be boolean, but not both.
|
Returns
|
the same type as parameters, float if one of parameters is float, otherwise int |
Examples
|
# Returns 3
REM(13, 5)
# Returns -3
REM(-13, 5)
|
Rotate
Creates whole SRT3D rotation property fields. You can use the SRT3D data type with the Layout Transformation and the Render Transformation properties. See RotateX, RotateY, RotateZ, CreateRotation, CreateRotationX, CreateRotationY, CreateRotationZ.
Syntax
|
Rotate(rotationField, value)
|
Parameters
|
rotationField
|
rotation property field of either the Layout Transformation or the Render Transformation property |
value
|
Vector3 |
|
Returns
|
quaternion |
Examples
|
# Rotates the bound node:
# - 40 degrees counterclockwise on the X axis
# - 80 degrees counterclockwise on the Y axis
# - 60 degrees counterclockwise on the Z axis.
Rotate({./Node3D.LayoutTransformation}.Rotation, Vector3(40, 80, 60))
|
RotateX
Creates whole SRT3D rotation property fieldss on the X axis. You can use the SRT3D data type with the Layout Transformation and the Render Transformation properties. See Rotate, CreateRotation, CreateRotationX, CreateRotationY, CreateRotationZ.
Syntax
|
RotateX(rotationField, value)
|
Parameters
|
rotationField
|
rotation property field of either the Layout Transformation or the Render Transformation property |
value
|
int, or float |
|
Returns
|
quaternion |
Examples
|
# Rotates the bound node 20 degrees counterclockwise on the X axis.
RotateX({./Node3D.LayoutTransformation}.Rotation, 20)
|
RotateY
Creates whole SRT3D rotation property fields on the Y axis. You can use the SRT3D data type with the Layout Transformation and the Render Transformation properties. See Rotate, CreateRotation, CreateRotationX, CreateRotationY, CreateRotationZ.
Syntax
|
RotateY(rotationField, value)
|
Parameters
|
rotationField
|
rotation property field of either the Layout Transformation or the Render Transformation property |
value
|
int, or float |
|
Returns
|
quaternion |
Examples
|
# Rotates the bound node 20 degrees counterclockwise on the Y axis.
RotateY({./Node3D.LayoutTransformation}.Rotation, 20)
|
RotateZ
Creates whole SRT3D rotation property fields on the Z axis. You can use the SRT3D data type with the Layout Transformation and the Render Transformation properties. See Rotate, CreateRotation, CreateRotationX, CreateRotationY, CreateRotationZ.
Syntax
|
RotateZ(rotationField, value)
|
Parameters
|
rotationField
|
rotation property field of either the Layout Transformation or the Render Transformation property |
value
|
int, or float |
|
Returns
|
quaternion |
Examples
|
# Rotates the bound node 20 degrees counterclockwise on the Z axis.
RotateZ({./Node3D.LayoutTransformation}.Rotation, 20)
|
Round
Calculates the closest integer.
Syntax
|
ROUND(value)
|
Parameters
|
value
|
color, int, float, Vector2, Vector3, Vector4: number to compute |
|
Returns
|
the same type as parameter |
Examples
|
# Returns 1.0
ROUND(0.8)
# Returns 2.0
ROUND(1.5)
# Returns 0.0
ROUND(0.1)
|
Square root
Calculates the square root of a number. The square root value of a number is always positive.
Syntax
|
SQRT(n)
|
Parameters
|
n
|
int, float, color, Vector2, Vector3, Vector4: number to compute |
|
Returns
|
the same type as parameter, except for int it returns float |
Examples
|
# Returns 5.0
SQRT(25)
|
Step
Compares a value to a threshold.
Syntax
|
STEP(threshold, value)
|
Parameters
|
threshold
|
int, float, color, Vector2, Vector3, Vector4: threshold to compare against |
value
|
int, float, color, Vector2, Vector3, Vector4: number to compute: the value to compare against the threshold |
|
Returns
|
float, 0.0 if the value is lower than the threshold , 1.0 if the value equals or is greater than the threshold |
Examples
|
# Returns 1.0
STEP(2, 5)
# Returns 0.0
STEP(0.0, -0.1)
# Returns 1.0
STEP(1.0, 1.0)
|
Common binding expressions
Constants
To bind a property value to a constant, enter just the constant.
# Binds the value of the selected property to 10.
10
Variables
In binding expressions you can use variables.
# Assigns the value 1 to the variable 'A', and binds the value of
# the variable to the selected property.
A = 1
# Same as above, but using an alternative syntax.
A = (1)
Property bindings
To bind a property to another property, enter in curly braces the relative path to the node which contains the property to which you want to bind, followed by a forward slash and the name of the source property.
When you use the @ sign before the path, Kanzi Studio updates the binding expression whenever the location between the source and the target nodes in the scene graph changes. Note that with the @ sign you can create bindings only within the same prefab, not between prefabs.
You can drag property names from the Properties to the Binding Argument Editor.
You can use the operators and parentheses with property values and property field values.
Syntax
|
{[path]/[property]}
|
Parameters
|
[path]
|
relative path to node |
[property]
|
name of the property |
|
Examples
|
# Binds to the Layout Width property of the current node.
{@./LayoutWidth}
# Binds to the FOV property of the Camera node.
{@../Camera/Fov}
# Same as above, but Kanzi Studio does not track the location of the target node.
{../Camera/Fov}
# Binds to the Vertical Margin property of the Box node.
{@../Box/LayoutVerticalMargin}
# Multiplies the FOV property with the Layout Transformation Scale X property field.
{@../Camera/Fov} * {../Box/LayoutTransformation}.ScaleX # Binds the Render Transformation Translation X and Translation Y property fields of
# the current node to each vector of a property which uses the Vector2D data type.
# In the Binding Argument Editor editor set the Property to a property which uses the
# Vector2D data type. For example, use the Horizontal Margin property.
X = {@./Node3D.RenderTransformation}.TranslationX
Y = {@./Node3D.RenderTransformation}.TranslationY
V = Vector2(0.0, 0.0)
V.VectorX = X
V.VectorY = Y
V
|
Prefab root bindings
A prefab can contain a tree of nodes, each with their own properties. When you edit the nodes in a prefab or any of its instances in a project, you change those nodes in all instances of that prefab. However, you can customize individual instances of the prefab to have individual values by overriding the values in the default prefab. For example, when you create a prefab for an address book entry you want to show a different name, number, and photo for each address book entry.
To bind a property of any node in a prefab to a property in the root of an instance of that prefab, in the binding expression enter in curly braces ##Template
, followed by the name of the property in the root of the prefab instance to which you want to bind.
For example, use the ##Template
binding syntax when you have a Text Block node in a Button prefab and you want to show different text in different instances of that prefab.
TIP
You can let Kanzi Studio create a prefab root binding for you. When you select any node in a prefab and in the Properties click
next to a property, Kanzi Studio:
- Creates from that property a custom property.
- Adds the custom property to the prefab and shows the custom property as a frequently used property in each instance of the prefab.
- Creates in the node the
##Template
binding to the custom property in the prefab root.
See Using bindings to customize instances of a prefab.
Syntax
|
{##Template/[property]}
|
Parameters
|
[property]
|
name of the property in the root of the prefab instance to which you want to bind |
|
Examples
|
# Binds to the MyProject.ContactNameText property in the root of the prefab instance.
{##Template/MyProject.ContactNameText}
|
Alias bindings
To bind a property to an alias, enter in curly braces the #
sign followed by the alias name, followed by a forward slash and property name of the item to which the alias points. See Using aliases.
Syntax
|
{#[aliasName]/[property]}
|
Parameters
|
[aliasName]
|
name of the alias |
[property]
|
name of the property |
|
Examples
|
# Binds to the Layout Width property of the target node of the alias named Sphere.
{#Sphere/LayoutWidth}
# Multiplies the FOV property of the target node of the alias named MainCamera
# with the Render Transformation property Scale X property field.
{#MainCamera/Fov} * {../Box/RenderTransformation}.ScaleX
|
Property field bindings
To bind a property to a property field of property, enter in curly braces the relative path to the node, followed by a forward slash and property name, followed by a period and the name of a property field. For property fields that are not common, instead of the property filed name use VectorN
, where N
is X
, Y
, Z
, or W
denoting the order in which the property is listed in the Properties. You can use the operators and parentheses with property values and property field values.
When you want to bind a property to a property field use these names.
Color property red color channel value |
ColorR |
Color_R, R |
Color property green color channel value |
ColorG |
Color_G, G |
Color property blue color channel value |
ColorB |
Color_B, B |
Color property alpha channel value |
ColorA |
Color_A, A |
Value of the node rotation around the X, Y, and Z axes which you set using rotation functions. See CreateRotation, CreateRotationX, CreateRotationY, CreateRotationZ, Rotate, RotateX, RotateY, and RotateZ. |
Rotation |
Rotation |
Value of the node rotation around the Z axis |
RotationZ |
Rotation_Z |
Value of the node scale along the X axis |
ScaleX |
Scale_X |
Value of the node scale along the Y axis |
ScaleY |
Scale_Y |
Value of the node scale along the Z axis |
ScaleZ |
Scale_Z |
Value of the node location along the X axis |
TranslationX |
Translation_X, X |
Value of the node location along the Y axis |
TranslationY |
Translation_Y, Y |
Value of the node location along the Z axis |
TranslationZ |
Translation_Z, Z |
The first property field of a property |
VectorX |
Vector_X |
The second property field of a property |
VectorY |
Vector_Y |
The third property field of a property |
VectorZ |
Vector_Z |
The fourth property field of a property |
VectorW |
Vector_W |
Syntax
|
{[path]/[property]}.[field]
|
Parameters
|
[path]
|
relative path to node |
[property]
|
name of the property |
[field]
|
name of the property field |
|
Examples
|
# Binds to the property field Scale X (value of the Scale X property field)
# of the Layout Transformation property of the Box node.
{../Box/LayoutTransformation}.ScaleX
# Binds to the property field Color R (value of the red color channel)
# of the Point Light Color property of the Point Light node.
{../Point Light/PointLightColor}.ColorR
# Light nodes have an attenuation property that has three property fields:
# Constant, Linear, and Quadratic.
# For example, for the Point Light:
# To bind to the first property field (Constant)
{../Light/PointLightAttenuation}.VectorX
# To bind to the second property field (Linear)
{../Light/PointLightAttenuation}.VectorY
# To bind to the third property field (Quadratic)
{../Light/PointLightAttenuation}.VectorZ
# Multiply property FOV with Render Transformation property Scale X property field.
{../Camera/Fov} * {../Box/RenderTransformation}.ScaleX # Binds to the Vertical Margin property Bottom property field of the Image node.
{@../Image/Node.VerticalMargin}.VectorX # Binds to the Vertical Margin property Top property field of the Image node.
{@../Image/Node.VerticalMargin}.VectorY # Binds the Render Transformation Translation X and Translation Y property fields of
# the current node to each vector of a property which uses the Vector2D data type.
# In the Binding Argument Editor editor set the Property to a property which uses the
# Vector2D data type. For example, use the Horizontal Margin property.
X = {@./Node3D.RenderTransformation}.TranslationX
Y = {@./Node3D.RenderTransformation}.TranslationY
V = Vector2(0.0, 0.0)
V.VectorX = X
V.VectorY = Y
V
|
Color property field bindings
Color4()
binds color property fields. Color4()
takes four arguments:
- The first specifies the value for the red color channel
- The second specifies the value for the green color channel
- The third specifies the value for the blue color channel
- The fourth specifies the value for the alpha channel.
Color values are mapped to the range 0..1.
Syntax
|
Color4(r, g, b, a)
|
Parameters
|
r
|
0...1 range: red color channel value
|
g
|
0...1 range: green color channel value
|
b
|
0...1 range: blue color channel value
|
a
|
0...1 range: alpha channel value
|
|
Examples
|
# Sets the color to white and opaque.
Color4(1, 1, 1, 1)
# Same as above, but with alternative syntax.
Color(1, 1, 1, 1)
# Sets the color to red with 50% transparency.
Color4(1, 0, 0, 0.5)
# Invalid expression, one argument is missing.
Color4(0.1, 1, 0.4)
# Use variables as property fields of the Color4() to assign the
# property field values of the whole Color property.
#
# Assigns custom properties Red, Green, and Blue to variables
# you use to control the color of a node.
red = {@./Red}
green = {@./Green}
blue = {@./Blue}
color = Color4(0, 0, 0, 1)
# Assigns the red, green, and blue variables to each color channel.
color.ColorR = red
color.ColorG = green
color.ColorB = blue
color
|
Data source bindings
To bind a data object to a property or a property field, enter in curly braces DataContext
followed by a period, followed by the data object to which you want to bind the property or property field. Use a period to access child data objects.
Syntax
|
{DataContext.DataObject}
|
Parameters
|
DataObject
|
The data object to which you want to bind. |
|
Examples
|
# Binds the property value of the item to the speed data object
# which is a child data object of the gauges data object
# in the data context of the item.
{DataContext.gauges.speed} # Binds the data context itself. For example, If you want to use the current data context
# as the property value.
{DataContext}
|
Grid Layout bindings
To set the size of columns and rows in a Grid Layout node using bindings, enter in quotation marks each value followed by a semicolon:
- Use a floating point number to specify that the size of a row or a column is fixed. This is the same as when you set the value of the Columns or Rows property in the Properties to Fixed and define the size of this column or row.
- Add an asterisk prefix to specify that the size of a row or a column is proportional. This is the same as when you set the value of the Columns or Rows property in the Properties to Proportional and define the proportion of the Grid Layout this column or row takes.
- Leave the definition empty to specify that the Grid Layout node automatically sets the size of a row or a column. This is the same as when you set the value of the Columns or Rows property in the Properties to Automatic.
Syntax
|
"value0;value1; ... valueN;"
|
Parameters
|
|
Examples
|
# This binding to the Columns property sets the width of each of the three columns to a fixed size:
# - The width of the first column is 2.0.
# - The width of the second column is 3.0.
# - The width of the third column is 4.0.
"2.0;3.0;4.0;"
# This binding to the Rows property sets the height of each of the three rows to the fixed size 5.0.
"5.0;5.0;5.0;" # This binding to the Rows property sets the height of the two rows to the height of their content.
";;" # This binding to the Columns property sets the width of the columns in relation to the total width of the Grid Layout node:
# - The width of the first column is 1/6 of the width of the Grid Layout node.
# - The width of the second column is 2/6 of the width of the Grid Layout node.
# - The width of the third column is 3/6 of the width of the Grid Layout node.
"*1.0;*2.0;*3.0;"
|
Transformation bindings
Use the Srt2D()
and Srt3D()
constants to transform the scale, rotation, and translation of the bound node.
Srt2D
Use Srt2D()
to apply transformation to a 2D node with the Render Transformation or Layout Transformation properties. In the Binding Argument Editor set the Property to Layout Transformation or Render Transformation.
Syntax
|
Srt2D(scaleX, scaleY, rotation, translationX, translationY)
|
Parameters
|
scaleX
|
int, float: the scale of the node on the x axis in percent |
scaleY
|
int, float: the scale of the node on the y axis in percent |
rotation
|
int, float: the rotation of the node in degrees |
translationX
|
int, float: the translation of the node on the x axis in pixels |
translationY
|
int, float: the translation of the node on the y axis in pixels |
|
Returns
|
Transformation2D, calculated transformation for the scale, rotation, and translation of the bound 2D node |
Examples
|
# Applies the transformation to the bound 2D node using the Layout Transformation or Render Transformation property,
# depending on which of these properties you set in the Binding Argument Editor:
# - Scales the node to 150% percent on both axes
# - Rotates the node clockwise 90 degrees
# - Translates the node 800 pixels on the x axis, and 60.5 pixels on the y axis.
Srt2D(1.5, 1.5, 90.0, 800.0, 60.5)
|
Srt3D
Use Srt3D()
to apply transformation to a 3D node with the Render Transformation or Layout Transformation properties. In the Binding Argument Editor set the Property to Layout Transformation or Render Transformation.
Syntax
|
Srt3D(scaleX, scaleY, scaleZ, rotationX, rotationY, rotationZ, translationX, translationY, translationZ)
|
Parameters
|
scaleX
|
int, float: the scale of the node on the x axis in percent |
scaleY
|
int, float: the scale of the node on the y axis in percent |
scaleZ
|
int, float: the scale of the node on the z axis in percent |
rotationX
|
int, float: the rotation of the node on the x axis in degrees |
rotationY
|
int, float: the rotation of the node on the y axis in degrees |
rotationZ
|
int, float: the rotation of the node on the z axis in degrees |
translationX
|
int, float: the translation of the node on the x axis in device independent units |
translationY
|
int, float: the translation of the node on the y axis in device independent units |
translationZ
|
int, float: the translation of the node on the z axis in device independent units |
|
Returns
|
Transformation3D, calculated transformation for the scale, rotation, and translation of the bound 3D node |
Examples
|
# Applies the transformation to the bound 3D node using the Layout Transformation or Render Transformation property,
# depending on which of these properties you set in the Binding Argument Editor:
# - Scales the node to 70% on all axes
# - Rotates the node counter-clockwise 30 degrees on the x axis, 60 degrees on the y axis, and 30 degrees on the z axis
# - Translates the node by 1 device independent unit on all axes
Srt3D(0.7, 0.7, 0.7, -30.0, -60.0, -30.0, 1.0, 1.0, 1.0)
|
See also
Using bindings
Using aliases
Troubleshooting bindings
Open topic with navigation