Customizing Android bindings¶
Setting up the environment¶
To set up the environment:
Set the environment variables on your computer:
Install Python 3.2 or newer and add the Python 3.x installation path to your system path. If you have Python 2.x installed, make sure that Python 3.x appears in your path before the Python 2.x.
Add Kanzi workspace to the
KANZI_HOME
environment variable.
Tip
To check that Python and Kanzi workspace are set, open the command line interface, type
set
, and press the Enter key. The environment variables are set if the output includes theKANZI_HOME
variable and thePATH
variable contains the path to the Python 3.2 installation directory.Install the Android SDK Build Tools 28 or newer.
In Android Studio open the
<KanziConnectInstallation>/SDK/bindings/android/AndroidGradleAarCreator
project.
Customizing the bindings AAR library¶
The binding_configuration.xml
file controls the generation of bindings. This file is included in the <KanziConnectInstallation>/SDK/bindings/android/AndroidGradleAarCreator
project.
In the AndroidGradleAarCreator project you can find the binding_configuration.xml
file in the Project files window in kanziconnect > kanziconnect > buildenv > configuration.
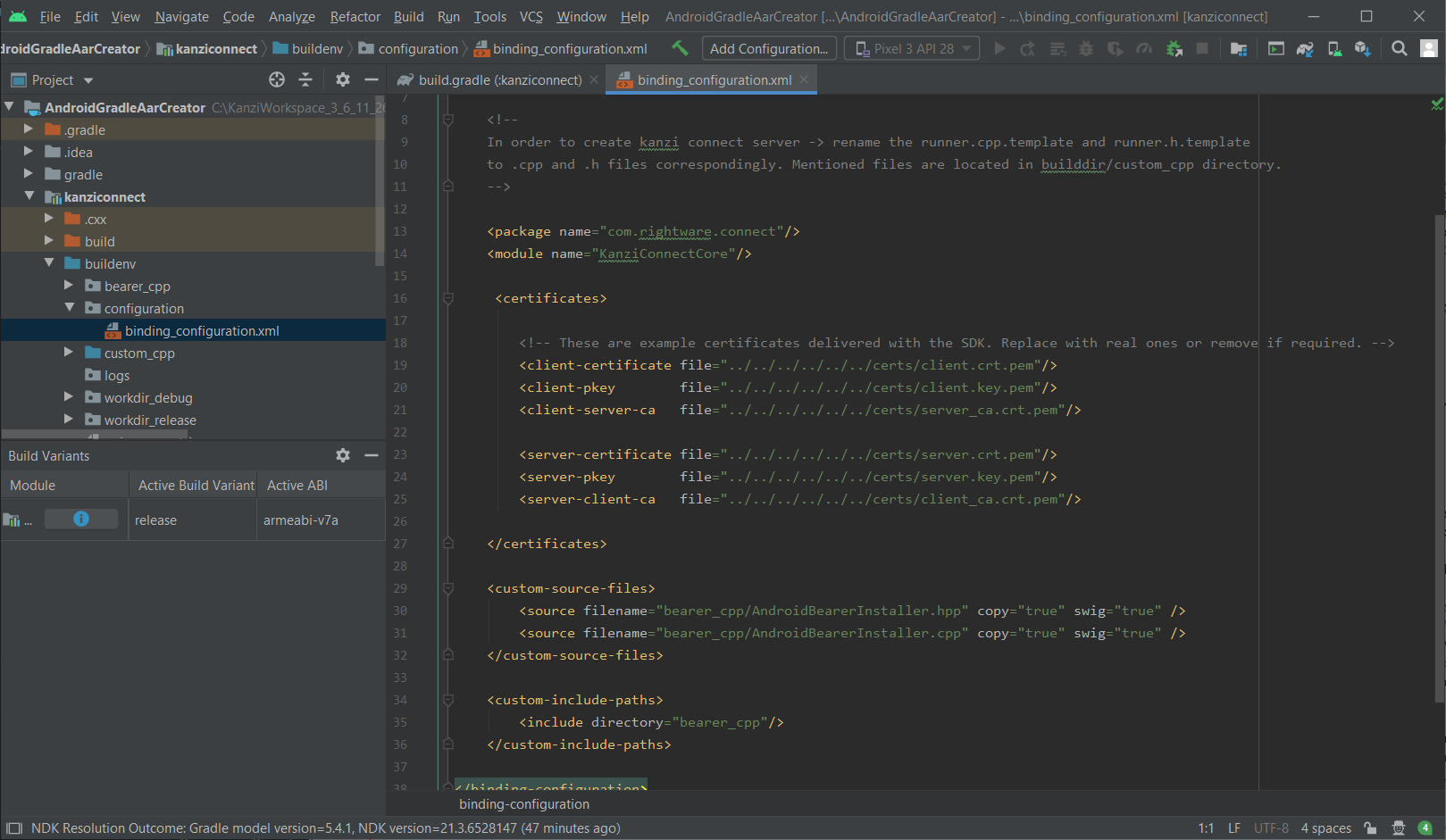
In the build_configuration.xml
you can:
Set whether the intermediate, generated .java and .cpp files are left after compilation. You can find the intermediate files in the
kanziconnect/buildenv/workdir_(release|debug)
directory.<keep-intermediate-files/>
Set the SSL certificates. The default certificates are the same as those which are used by the native Kanzi Connect applications so that the binding components can communicate with then using SSL.
<certificates> <!-- SDK contains these example certificates. Replace with real certificates, or remove. --> <client-certificate file="../../../../../../certs/client.crt.pem"/> <client-pkey file="../../../../../../certs/client.key.pem"/> <client-server-ca file="../../../../../../certs/server_ca.crt.pem"/> <server-certificate file="../../../../../../certs/server.crt.pem"/> <server-pkey file="../../../../../../certs/client.key.pem"/> <server-client-ca file="../../../../../../certs/client_ca.crt.pem"/> </certificates>
Define which C++ files must be compiled in the bindings library. Special handling is reserved for files named
runner.cpp
andrunner.h
with this definition inside the<custom-source-files>
:<source file-name="../../../../../examples/AndroidServer/configs/platforms/android/jni_src/runner.h" copy="true" /> <source file-name="../../../../../examples/AndroidServer/configs/platforms/android/jni_src/runner.cpp" copy="true"/>
The referenced
runner.cpp
andrunner.h
are temporarily copied from specified source location and are run through SWIG so that the classes defined in these files are accessible from the Java code. These files implement custom server runner when implementing the Java-based Kanzi Connect Server.This approach is used in the
<KanziConnectInstallation>/SDK/examples/AndroidServer/configs/platforms/android/binding_instructions.xml
and related runner source files are in the<KanziConnectInstallation>/SDK/examples/AndroidServer/configs/platforms/android/jni_src/
directory.Use this example as a starting point for creating your own Java-based Kanzi Connect Server.
<custom-source-files> <source filename="../../../../../sources/myfile.cpp "/> <source filename="../../../../../sources/myfile.hpp "/> </custom-source-files>
Define the header include paths that are searched during the compilation. Use this setting when you use custom sources.
<custom-include-paths> <include directory="../../../../../sources/"/> </custom-include-paths>
Do not change the module name for the generated code.
<module name="KanziConnectCore"/>
Do not chnage the setting that sets the name of the package for the generated Java classes.
<package name="com.rightware.connect"/>
Building the AAR library package¶
After you set up the environment, you can build the AAR library package. Build the AAR library package when you must recreate the Android bindings.
To build the release AAR library package, select Build > Select Build Variant, in the Build Variants window select release, and then Build > Rebuild Project.
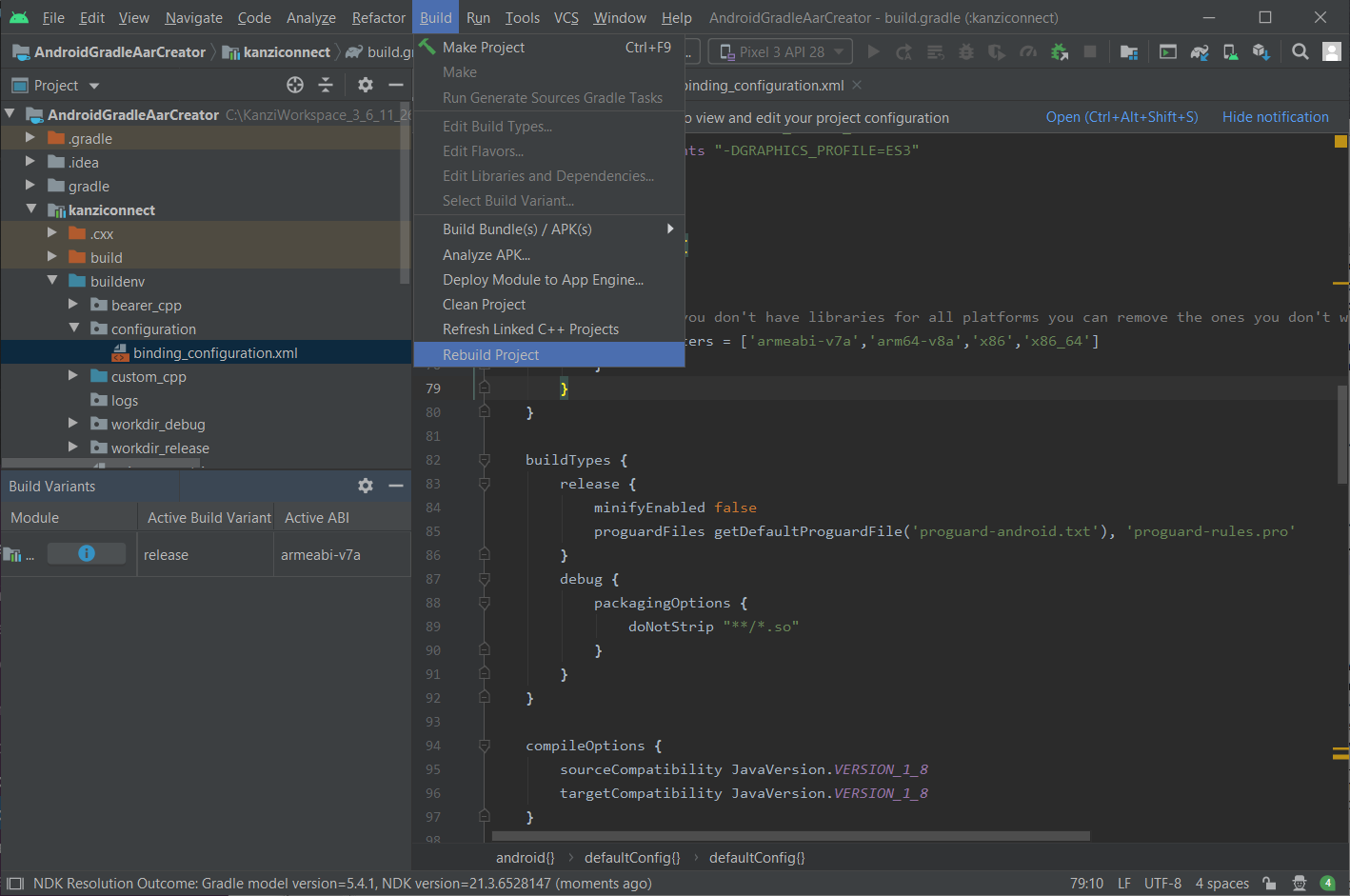
Note
Android Studio does not support specific ABI builds in the Build Variant configuration. However, in build.gradle you can remove the ABI builds that you do not need.
For example, in the Build Variant window even if you select armeabi-v7a, Android Studio builds all ABIs (‘armeabi-v7a’,’arm64-v8a’,’x86’,’x86_64’).
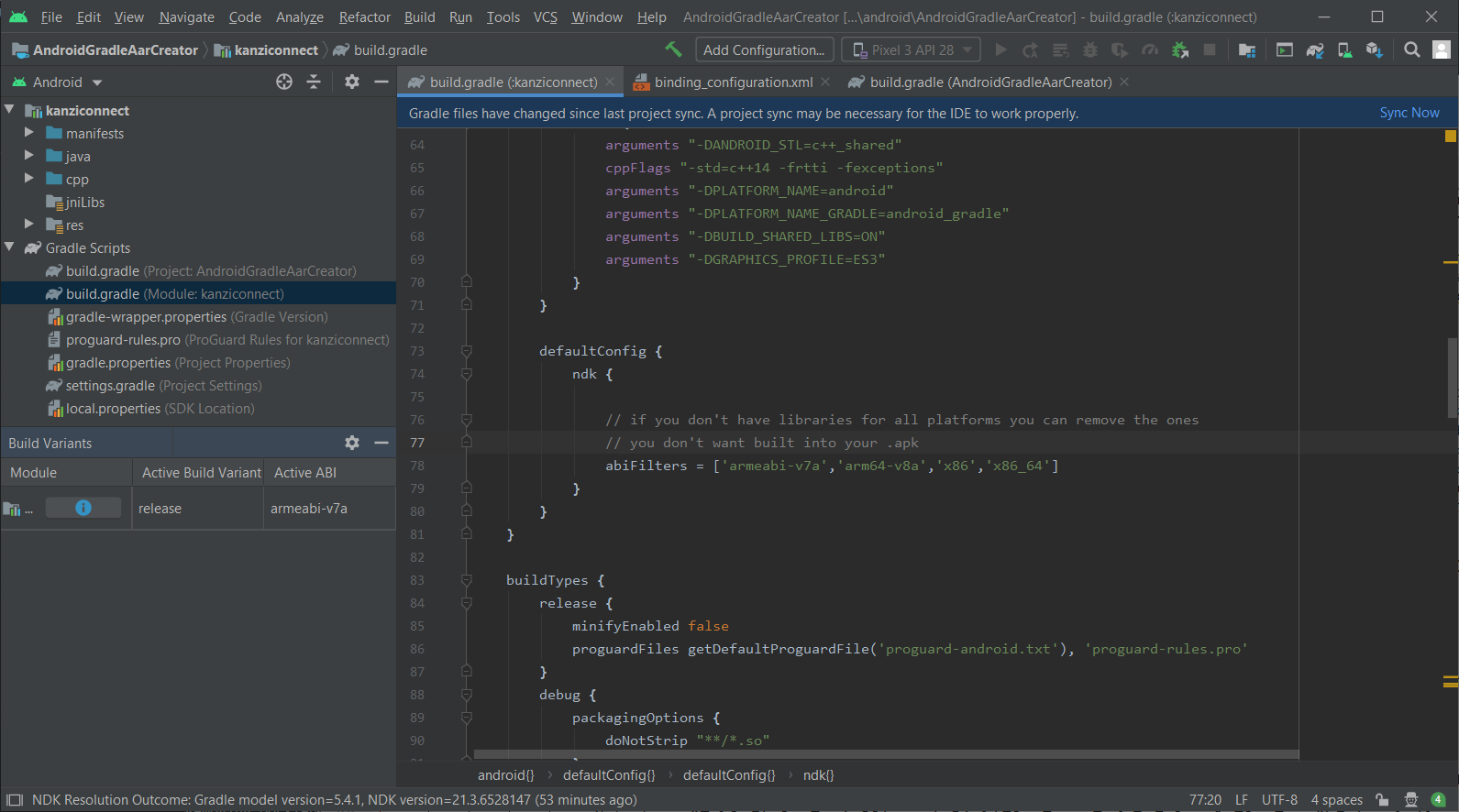
When the compilation is successful, you can find the generated kanziconnect-release.aar
in the <KanziConnectInstallation>/SDK/bindings/android/AndroidGradleAarCreator/kanziconnect/build/outputs/aar
directory. You can use this AAR package in your Kanzi Connect application. See Creating an Android application that uses Kanzi Connect bindings.
Utility classes provided with Kanzi Connect Android bindings¶
These files are included in the <KanziConnectInstallation>/SDK/bindings/android/AndroidGradleAarCreator
project as source files and you can edit them so that they suit your purpose. These files are always included in the generated AAR files.
File name |
Description |
---|---|
|
Java implementation to read the standard Kanzi Connect |
|
Use this helper class to integrate Kanzi Connect functionality to UI of an Android application. Capable of running one remote service and any number of client instances. |
|
Use this helper class to run Kanzi Connect functionality in a dedicated worker thread. You can use this when you need functionality on a headless process. Capable of running one remote service and any number of client instances. |