Troubleshooting the performance of your application¶
Kanzi provides different means of measuring the performance of your application and displays various performance parameters. Use these means and parameters to improve the performance of your application.
Viewing the application performance in Kanzi Studio¶
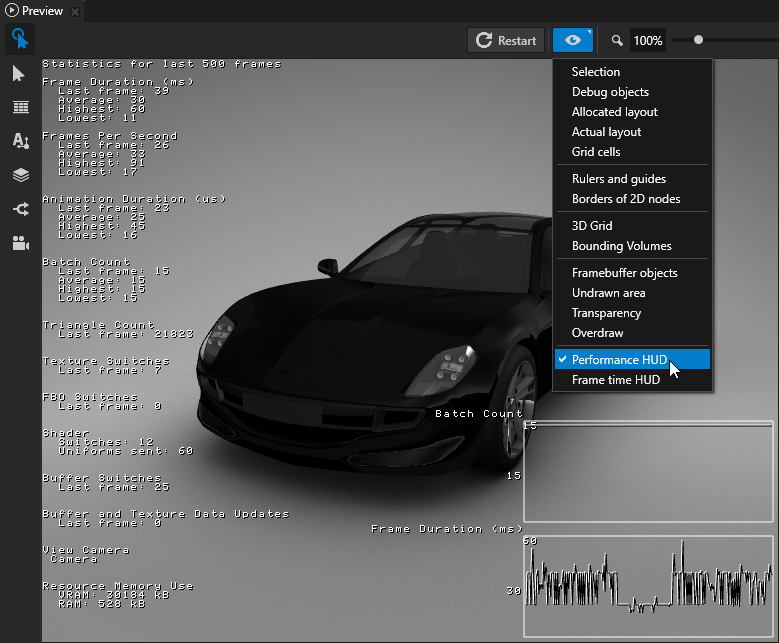
To analyze the performance and inner structure of your application in Kanzi Studio, in the Preview click to enter the Analyze mode, right-click
, and select:
Performance HUD shows the overall performance indicators of your Kanzi application during the last 500 frames including:
Frame Duration (ms) shows the time in milliseconds it took to render the last frame, and the average, highest, and lowest times.
The top value in the Frame Duration (ms) graph shows the longest, and the bottom value the average time it took to render a frame during the last 500 frames.
Use this information to identify performance bottlenecks in your Kanzi application. For example, you can use it to find out how to improve the performance of your application by distributing rendering across several frames. See Distributing rendering across several frames.
Frames Per Second shows the number of frames rendered per second.
Animation Duration (μs) shows the time in microseconds it takes to execute all animations in the application for the last frame, and the average, highest, and lowest times.
Batch Count shows how many individual draw calls were executed (
glDrawElements
andglDrawArrays
).You can access the batch count using the Kanzi Engine API by calling
getBatchCount()
.The top value in the Batch Count graph shows the highest number of batches during the last 500 frames, and the bottom value the number of batches in the last frame.
Triangle Count shows how many individual triangles were drawn in total during a frame.
You can access the triangle count in the Kanzi Engine API by calling
getTriangleCount()
.Texture Switches shows how many times a new texture was bound to GPU.
You can access the texture switch count in the Kanzi Engine API by calling
getTextureSwitchCount()
.FBO Switches shows how many framebuffer objects were bound to GPU. See Rendering best practices.
You can access the buffer switch count in the Kanzi Engine API by calling
getFramebufferSwitchCount()
.Shader shows how many times a new shader was bound for the newly applied batch and how many individual shader uniforms were sent for the last frame. See Reducing shader switches.
You can access the shader switch count in the Kanzi Engine API by calling
getShaderSwitchCount()
.You can access the uniforms sent in the Kanzi Engine API by calling
getUniformSendCount()
.Buffer Switches shows how many vertex, index, and uniform buffer objects were bound to GPU.
You can access the buffer switch count in the Kanzi Engine API by calling
getBufferSwitchCount()
.Buffer and Texture Data Updates shows how many times new vertex buffer or texture data was uploaded to the GPU. If the same buffer is accessed for write and read operations, these heavyweight operations can cause delay in GPU execution. The ideal value is 0.
For example, this value can increase when the text glyph cache needs frequent updates. In this case, to decrease glyph cache updates, reduce the value of the Font Size property in Text Block nodes.
You can access this in the Kanzi Engine API by calling
getHeavyweightCallCount()
.View Camera shows the name of the Camera node that is used for displaying the scene in your application.
Resource Memory Use shows an estimated amount of local GPU memory (VRAM) and non-local GPU memory (RAM) that your Kanzi application uses.
The values that the Performance HUD shows in the Kanzi Studio Preview and when you run the Kanzi application on a target device differ because Kanzi Studio loads and keeps in memory all resources in the application.
Timer subscriptions shows the number of registered timer handlers in the
MessageDispatcher
. Too many timer handlers can decrease the performance of your application.You can access the number of timer subscriptions in the Kanzi Engine API by calling
getSubscriptionCount()
.Animations shows the number of active and all animations in your Kanzi application.
You can access the number of animations in the Kanzi Engine API:
To get the number of active animations, use
getActiveTimelinePlaybackCount()
.To get the number of all animations, use
getTimelinePlaybackCount()
.
Framebuffer objects marks with translucent yellow moving stripes node which are rendered into textures and cause a framebuffer object switch.
Transparency marks transparent nodes with translucent blue moving stripes. See Configuring nodes for efficient rendering.
Overdraw visualization indicates areas where Kanzi renders multiple times to the same pixel. Lighter green color indicates higher amount of overdraw.
Viewing the overall performance¶
To view the overall performance of your Kanzi application:
In the Kanzi Studio Preview, click
and in the context menu enable Performance HUD.
In the
application.cfg
, enable the display of the full Performance HUD:PerformanceInfoLevel = 2
See PerformanceInfoLevel.
Enable the display of the Performance HUD in the application code in the
onConfigure()
functionconfiguration.performanceInfoLevel = ApplicationProperties::PerformanceInfoLevelFull;
See PerformanceInfoLevel.
In the
application.hpp
, enable the display of Performance HUD by calling thegetPerformanceInfoLevel()
andsetPerformanceInfoLevel()
functions:ApplicationProperties::PerformanceInfoLevel getPerformanceInfoLevel() const { return m_applicationProperties.performanceInfoLevel; } void setPerformanceInfoLevel(ApplicationProperties::PerformanceInfoLevel level) { m_applicationProperties.performanceInfoLevel = level; }
To write the frame rate values to a log file, you can retrieve the current frame rate value from the Kanzi Engine by calling the
getFramesPerSecond()
function in theapplication.hpp
. You can retrieve Kanzi Engine from the application by callinggetEngine()
.To get the amount of time it takes to render a frame, use the
MainLoopScheduler
timing interfacegetLastFrameDuration()
.
Viewing the loading times of resources¶
In the application.cfg
file or in the application code in the onConfigure()
function you can set Kanzi to print to the debug console the amount of time it takes to load the resources kzb files that your application uses. See Measuring the loading and deployment time of resources.
Viewing the graphics performance¶
In the application.cfg
file or in the application code in the onConfigure()
function you can set Kanzi to print to the debug console:
Graphics-related information on application startup, such as the GL renderer, version, and shading language version. See LogOpenGLInformation.
List of graphics-related extensions on application startup. See LogOpenGLExtensions.
List of graphics-related properties on application startup, such as swap behavior, display size, and your application window size. See LogSurfaceInformation.
Log of the graphics API calls of your application. See GraphicsLoggingEnabled.
Viewing the overdraw of nodes¶
Overdraw visualization indicates areas where Kanzi renders multiple times to the same pixel. Lighter green color indicates higher amount of overdraw.
Kanzi uses the stencil buffer to calculate how many times it draws to the same pixel. If you are using the stencil buffer to render your content, the Overdraw visualization can return unexpected results.
The overdraw visualization does not show:
Overdraw for 3D nodes that you render to a composition, which you then render to a texture. In that case, the Overdraw visualization shows the content from a composition that you render to a texture, as a single quad.
Parts of the nodes that are clipped when you set in their ascendant node the Clip Children property to enabled.
Nodes that are completely translucent or nodes that are not visible, including their descendant nodes.
To view overdraw in your application, in the Kanzi Studio Preview click to enter the Analyze mode, right-click
, and select Overdraw.
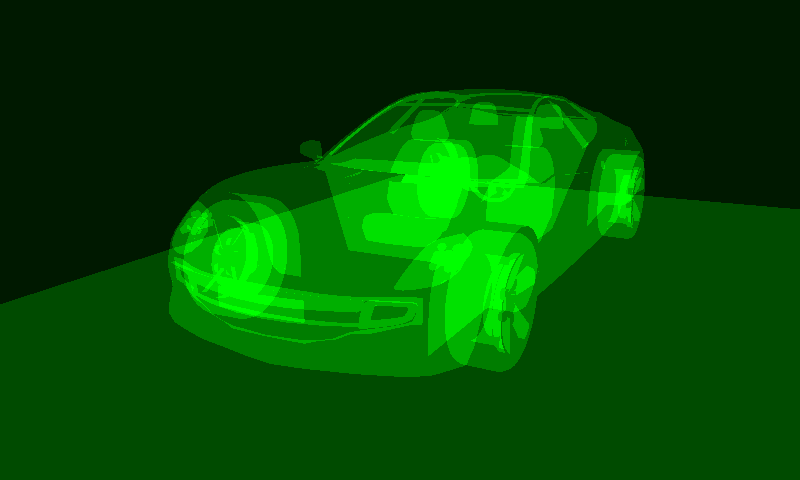
Viewing nodes that are rendered into texture¶
Switching between framebuffer objects can cause significant performance reduction on some platforms. Conditions when Kanzi renders a node into a texture can be complex. For example, rotation, scale, or opacity can cause render to texture to occur.
To see whether a node is rendered into a texture, and causing a framebuffer object switch, in the Preview click to enter the Analyze mode, right-click
, and select Framebuffer objects.
The Preview highlights the layers that are rendered into texture with transparent, orange stripes.
Viewing nodes rendered to a composition render target¶
In Kanzi Studio you can see which 2D nodes in your application Kanzi renders to a composition render target.
To view in the Preview the 2D nodes that use alpha blending in the Preview click to enter the Analyze mode, right-click
, and select Transparency.
The Preview highlights the nodes that Kanzi renders to a composition render target with translucent, blue stripes.