Using plugins in Kanzi Android framework (droidfw)¶
Using Kanzi Engine Java plugins¶
To learn about creating Kanzi Engine Java plugins, see Creating Kanzi Engine Java plugins.
To use a plugin in your Android application, either:
Load the plugin during the initialization of an Activity.
@Override protected void onCreate(Bundle icicle) { ... KanziRuntime.Reference runtimeRef = KanziRuntime.acquire(this); runtimeRef.get().getDomain().registerPlugin(new MyPlugin()); ... }
override fun onCreate(icicle: Bundle) { ... val runtimeRef = KanziRuntime.acquire(this) runtimeRef.get().domain.registerPlugin(new MyPlugin()); ... }
Load the plugin when your application attaches a Kanzi view that uses the plugin.
view.addListener(new KanziViewListener() { ... @Override public void onAttachedToWindow(View view, Domain domain) { domain.registerPlugin(new MyPlugin()); ... } ... }
view.addListener(object : KanziViewListener { ... override fun onAttachedToWindow(view: View, domain: Domain) { domain.registerPlugin(MyPlugin()) ... } ... }
Using Kanzi Studio generated plugins¶
Kanzi Studio automatically generates plugins for some features, such as Code Behind, and adds the registration for these plugins to the KanziGenerated.java
file.
To use these plugins, during the initialization of your activities or the attachment of your Kanzi views, call KanziGenerated.registerPlugins(domain)
. This is similar to the registration of individual plugins in Using Kanzi Engine Java plugins.
Using Kanzi Engine C++ plugins¶
To use a Kanzi Engine C++ plugin:
Export the Kanzi Engine plugin:
In Kanzi Studio open the project that contains the plugin code.
For example, open
<KanziWorkspace>/Tutorials/Data sources/Completed/Tool_project/XML data source.kzproj
.In the Library > Build Configurations select the plugin and in the Properties set:
CPU Architecture to match the architecture of your device
Build Profile to the configuration that you want to use
Select File > Export > Build Android Package.
Kanzi Studio builds the Kanzi Engine plugin in
<ProjectName>/Application/output/cmake/<PluginProjectName>/cmake/release/arm64-v8a/lib<PluginProjectName>.so
.For example, if you use the XML data source plugin, Kanzi Studio builds the plugin in
<KanziWorkspace>/Tutorials/Data sources/Completed/Application/output/cmake/XML_data_source/cmake/release/arm64-v8a/libXML_data_source.so
.
Add the built Kanzi Engine plugin to an application:
In Kanzi Studio select File > Close All projects and open or create a Kanzi Studio project using the Android application template.
Android application template creates a Kanzi Studio project with a Kanzi Android framework-based application.
In the Library right-click Kanzi Engine Plugin, select Import Kanzi Engine Plugin, and select the dll of the built plugin.
For example, select the
<KanziWorkspace>/Tutorials/Data sources/Completed/Application/lib/Win64/GL_vs2019_Release_DLL/XML_data_source.dll
plugin.This way you add Kanzi Engine plugin to a Kanzi Studio project.
In File Explorer in the project to which you want to add this Kanzi Engine plugin, create this directory structure
<ProjectName>/Application/lib/Android/release/arm64-v8a
.Copy the Kanzi Engine so plugin file from
<ProjectName>/Application/output/cmake/<PluginProjectName>/cmake/release/arm64-v8a/lib<PluginProjectName>.so
to the<ProjectName>/Application/lib/Android/release/arm64-v8a
directory.For example, copy the
<KanziWorkspace>/Tutorials/Data sources/Completed/Application/output/cmake/XML_data_source/cmake/release/arm64-v8a/libXML_data_source.so
file.Edit the
<ProjectName>/Application/CMakeLists.txt
file to add the Kanzi Engine plugin as an imported library dependency:add_library(<PluginProjectName> SHARED IMPORTED) set_target_properties(<PluginProjectName> PROPERTIES IMPORTED_LOCATION "${PROJECT_SOURCE_DIR}/lib/Android/release/${ANDROID_ABI}/lib<PluginProjectName>.so") target_link_libraries(<ProjectName> <PluginProjectName>)
For example, to add the XML data source plugin, use:
add_library(XML_data_source SHARED IMPORTED) set_target_properties(XML_data_source PROPERTIES IMPORTED_LOCATION "${PROJECT_SOURCE_DIR}/lib/Android/release/${ANDROID_ABI}/libXML_data_source.so") target_link_libraries(<ProjectName> XML_data_source)
This way you add the built native Kanzi Engine plugin to your Android application.
With this CMake configuration, you link a dynamically linked Kanzi Engine plugin to your Android application during build time. This way, when you add to your application a kzb file with a plugin dependency, Kanzi is able to load that plugin.
In Kanzi Studio select File > Export > Build Android Package.
Kanzi Studio builds and deploys your application to an Android device. When application starts running on your Android device, in the logcat you can see when the application loads the plugin.
For example, if you use the XML data source plugin, the logcat shows:
I/Kanzi: [info:generic] Loading plugin 'libXML_data_source.so'
Use the plugin in the Kanzi Android framework (droidfw) application.
For example, to learn how to use a Kanzi Engine data source plugin, see Using a data source.
Moving Kanzi Engine C++ plugin code to your application project¶
You can add a Kanzi Engine C++ plugin to a Kanzi Android framework (droidfw) application project. After this, you can merge the C++ plugin code with the application code. This way, you can build both the plugin and application in a single build from source.
To move Kanzi Engine C++ plugin code to your Kanzi Android framework (droidfw) application project:
(Optional) To ensure that the relative paths given in these instructions work, make a copy of your Kanzi Android framework (droidfw) application in the
<KanziWorkspace>/Projects
directory.Copy the plugin C++ code and its
CMakeLists.txt
to the<ProjectName>/Application/src/plugin
directory.For example, to add the XML Data Source plugin code to the project, copy the content of the
<KanziWorkspace>/Tutorials/Data sources/Completed/Application/src/plugin
directory to the<KanziWorkspace>/Projects/MyProject/Application/src/plugin
directory.The XML Data Source plugin allows a Kanzi application to consume data from an XML file. To learn how to define an XML data source, see Tutorial: Get application data from a data source.
In the
<ProjectName>/Application/src/plugin/CMakeLists.txt
file, change the path to the Kanzi build scripts.For example, change
if(NOT KANZI_ENGINE_BUILD) find_package(Kanzi REQUIRED CONFIG CMAKE_FIND_ROOT_PATH_BOTH HINTS "../../../../../../Engine/lib/cmake/Kanzi") endif()
to
if(NOT KANZI_ENGINE_BUILD) find_package(Kanzi REQUIRED CONFIG CMAKE_FIND_ROOT_PATH_BOTH HINTS "../../../../../Engine/lib/cmake/Kanzi") endif()
Move the C++ code and
CMakeLists.txt
file of your application to the<ProjectName>/Application/src/executable
directory.For example, in the
<KanziWorkspace>/Projects/MyProject/Application/
directory, move:CMakeLists.txt
to thesrc/executable
directorysrc/myproject.cpp
to thesrc/executable/src
directory
In the
<ProjectName>/Application/src/executable/CMakeLists.txt
file:Replace all occurrences of the project name with a new name.
For example, change
project(MyProject)
to
project(MyProject_executable)
Edit the path to the Kanzi build scripts. Change
include(${CMAKE_CURRENT_LIST_DIR}/cmake/kanzi-locate.cmake)
to
include(${CMAKE_CURRENT_LIST_DIR}/../../cmake/kanzi-locate.cmake)
Update the path to the plugin shared object.
For example:
add_library(XML_data_source SHARED IMPORTED) set_target_properties(XML_data_source PROPERTIES IMPORTED_LOCATION "${CMAKE_CURRENT_LIST_DIR}/../../lib/android/${CMAKE_BUILD_TYPE}/${ANDROID_ABI}/libXML_data_source.so") target_link_libraries(MyProject_executable XML_data_source)
In the
<ProjectName>/Application/
directory, create aCMakeLists.txt
file that is common for the entire project.For example:
cmake_minimum_required(VERSION 3.5.1) project(MyProject) add_subdirectory(src/plugin) add_subdirectory(src/executable)
In the
<ProjectName>/Application/configs/platforms/android_gradle/
directory, create a directory for the plugin project.For example, copy the
<KanziWorkspace>/Tutorials/Data sources/Completed/Application/configs/platforms/android_gradle/XML_data_source
directory to the<KanziWorkspace>/Projects/MyProject/Application/configs/platforms/android_gradle/
directory.In the
<ProjectName>/Application/configs/platforms/android_gradle/app/build.gradle
file:Change
externalNativeBuild { cmake { path file("$rootDir/../../../CMakeLists.txt") } }
to
externalNativeBuild { cmake { path file("$rootDir/../../../src/executable/CMakeLists.txt") } }
Because the application project depends on the plugin project, CMake must build the plugin code first. To set the build order, add the
afterEvaluate
block.For example:
afterEvaluate { // Make the CMake configuration task of this app depend on the build results of the // plugin project. tasks.withType(com.android.build.gradle.tasks.ExternalNativeBuildJsonTask).every { task -> // This example dependency assumes that the plugin project and application // project have the same build variant types available. // For example, :app:Debug -> :XML_data_source:Debug. task.dependsOn(":XML_data_source:externalNativeBuild${task.variantName.capitalize()}") } }
In the
dependencies
block, add a dependency on the plugin project.For example:
dependencies { implementation project(':XML_data_source') // ... }
In the
<ProjectName>/Application/configs/platforms/android_gradle/settings.gradle
file, include the name of the plugin project.For example:
rootProject.name = "myproject" include ":app" include ":XML_data_source"
Now, you can simultaneously edit and build both the Kanzi application and the C++ plugin code in Android Studio.
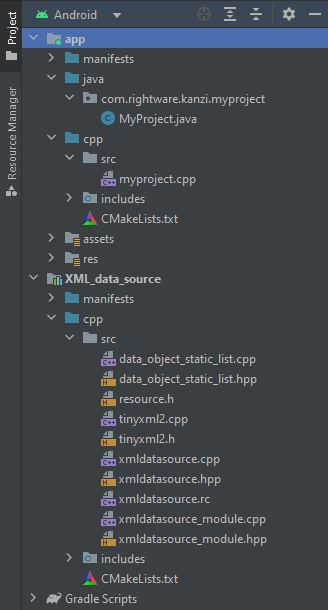
To use the updated Kanzi Engine plugin in Kanzi Studio:
Build the Kanzi Engine plugin DLL. See Creating a native Kanzi Engine plugin using a template.
In Kanzi Studio, update the Kanzi Engine plugin. See Updating a Kanzi Engine plugin in a project.